Framework information
Loadero supports writing the test script in the following frameworks to simulate participant actions:
You will be prompted to select one of these frameworks when creating a project.
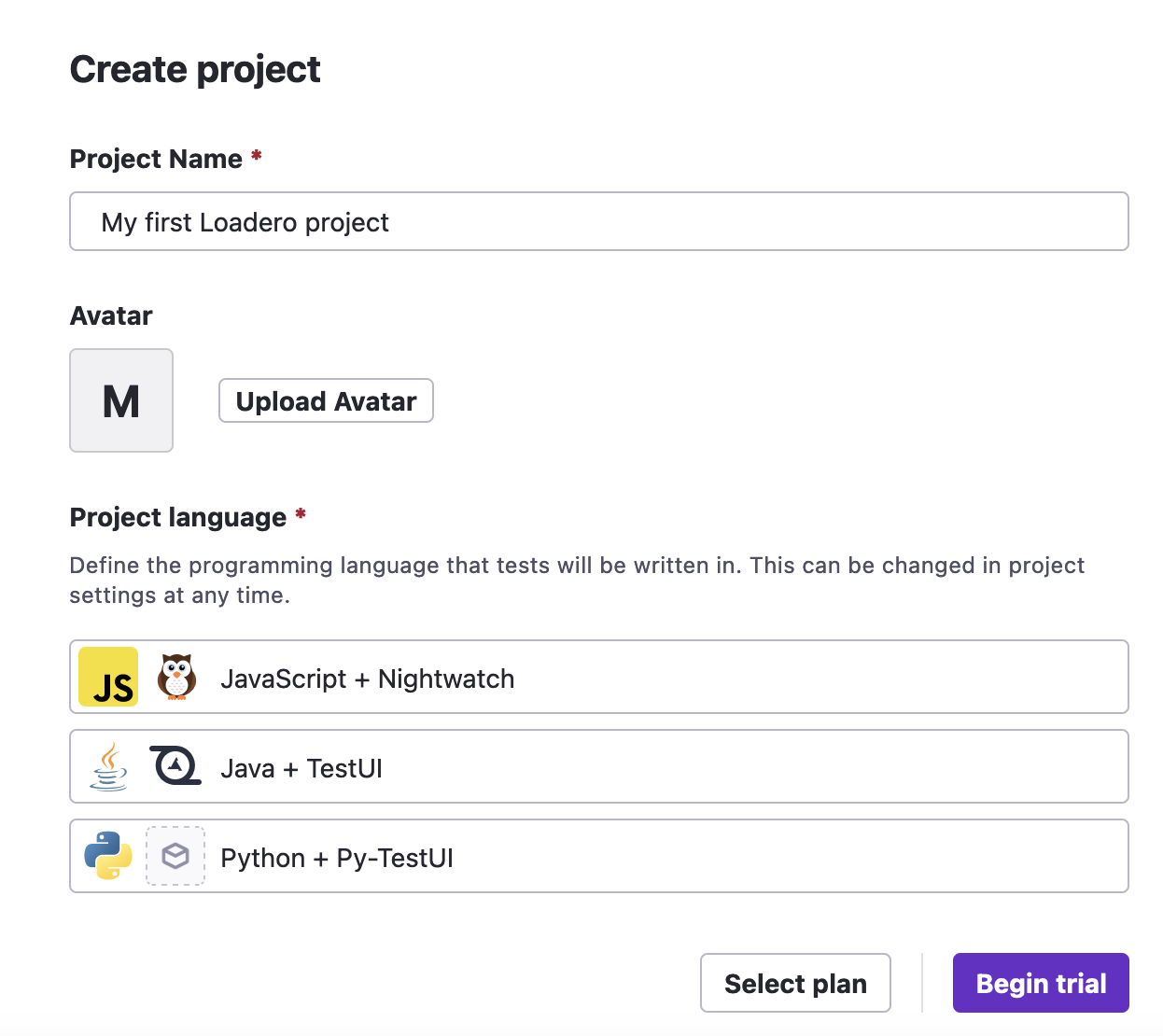
The project language can later be changed any time in the project settings.
Project language is a project-wide setting, it is not test-specific. If you have set the project language to be JavaScript + Nightwatch.js, and create a test with a Java + TestUI script, then when executing the test, the run will fail horribly due to perceived syntax errors, as the test will be attempted to be executed as a JavaScript + Nightwatch.js test.
It is possible, but not recommended, to have tests of different languages in one project, as you would have to switch the project language to the necessary one before launching a test every time.
In addition to the built-in commands in these frameworks, the Loadero team has implemented multiple additional custom commands that are at the user's disposal.
Available third party tools
Additional tools are pre-installed and available for use in the script. You do not have to add any import statements to the script, these imports are already handled in the background. Select the tab of the framework you are interested in to see the relevant tools.
- JavaScript + Nightwatch.js
- Java + TestUI
- Python + Py-TestUI
When scripting in Nightwatch.js, you will have access to the following tools:
- Node - v14.18.3
- The crypto module is pre-imported and can be used immediately.
- Other built-in Node modules have to be imported manually within the script (example provided below).
- The following NPM (v6.14.15)
packages come pre-imported and can be used immediately. NPM packages not
listed here will not be available for use within the script.
- jsonwebtoken as
jwt
- v8.5.1 - axios - v0.27.2
- moment - v2.29.4
- websocket-as-promised - v2.0.1
- websocket - 1.0.34
- jsonwebtoken as
client => {
// No import required, axios can be called immediately
axios
.get("https://petstore.swagger.io/v2/user/login?username=randomUser&password=superSecure")
.then((response) => {
console.log("API Response:", response.data)
});
// A pause to keep the test running long enough for the logging to complete
client
.pause(5*1000);
}
client => {
// Import the url module from Node.js
const url = require('url');
client
.url("https://bing.com")
.getCurrentUrl((result) => {
console.log("Current URL:", result.value);
// Parse the URL using Node's 'url' module
const parsedUrl = url.parse(result.value);
// Log parts of the parsed URL
console.log("Protocol:", parsedUrl.protocol);
console.log("Host:", parsedUrl.host);
console.log("Pathname:", parsedUrl.pathname);
console.log("Params:", parsedUrl.search)
// Identify each individual param
const params = parsedUrl.search.substring(1).split("&");
// Assert that params equal/match expected values
client.assert.strictEqual(params[0], "toWww=1", "toWww param is equal to 1.");
client.assert.match(params[1], /redig/), "redig param has a value";
});
}
Tests written in TestUI will be executed within the context of an environment
using Maven v3.9.0 and
Java v11.0.18. The following Java packages are
available for use within the script immediately without the use of import
statements:
- java.io.*
- java.util.*
- java.net.*
- java.net.http.*
- java.nio.file
- Files
- Path
- Paths
- org.json.simple.* - v1.1.1
- parser.*
- org.yaml.snakeyaml.Yaml - v2.2.0
- com.auth0.jwt - v4.0.0
- algorithms.*
- exceptions.*
- interfaces.*
- impl.*
Refer to the script at the end of the next section for an example on how to utilize imports in a Loadero script.
Tests written in Py-TestUI will be executed using
Python v3.12.3. The following Python packages
are available for use within the script immediately without the use of import
statements:
- datetime
- uuid
- requests - v2.28.1
- jwt - v2.4.0
- websockets - v10.3
- asyncio - v3.4.3
selenium.webdriver.common.keys.Keys
from selenium - v.4.22.0
Refer to the script at the end of the next section for an example on how to utilize imports in a Loadero script.
If you require access to other packages/imports, reach out to our team and we will see if we can make it available within the Loadero environment.
Available imports from the framework
While the section above details third-party tools, this section lists
framework-specific imports that you are able to use in your scripts. These
imports are available immediately and do not need to be imported via an
import
statement within the test script.
- JavaScript + Nightwatch.js
- Java + TestUI
- Python + Py-TestUI
There are no imports available from Nightwatch.js.
You can use the following imports from TestUI:
- Non-static
testUI.Configuration;
testUI.Utils.TestUIException
testUI.collections.UICollection
testUI.elements.UIElement
testUI.BrowserLogs
- Static
testUI.UIOpen.open
testUI.UIOpen.navigate
testUI.UIUtils.putLog
testUI.UIUtils.executeJs
testUI.Utils.AppiumHelps.sleep
testUI.Utils.By.*
testUI.elements.TestUI.E
testUI.collections.TestUI.EE
testUI.TestUIDriver.getSelenideDriver
testUI.TestUIDriver.setDriver
public void testUIWithLoadero() {
open("https://bing.com/");
E(byCssSelector("[type=search]")).waitFor(10).untilIsVisible();
// Using the static testUI.testUIDriver.getSelenideDriver import from TestUI
String pageTitle = getSelenideDriver().getTitle();
String pageURL = getSelenideDriver().getCurrentUrl();
// Using the java.util.* import from Java
Map<String, String> pageData = new HashMap<>();
pageData.put("title", pageTitle);
pageData.put("url", pageURL);
// Using the org.json.* import from Java
JSONObject jsonObject = new JSONObject(pageData);
System.out.println("Captured page data in JSON format: ");
System.out.println(jsonObject.toString());
}
You can use the following imports from Py-TestUI:
testui.support.testui_driver.TestUIDriver
testui.support.logger
testui.elements.testui_element.e
testui.elements.testui_collection.ee
def test_on_loadero(driver: TestUIDriver):
driver.navigate_to("https://www.bing.com")
# Using the testui.elements.testui_element.e import
e(driver, "css", "[type=search]").wait_until_visible(seconds=10)
# Using the uuid import
uuid_value = str(uuid.uuid4())
print(uuid_value)
e(driver, "css", "[type=search]").send_keys(uuid_value)
driver.save_screenshot("uuid_typed.png")